
Unit Testing 101: From Zero to Hero
30 Aug 2021 #tutorial #csharpDo you want to start writing unit tests, but don’t know where to start? Do you want to adopt unit testing in your team? I can help you!
If you’re a beginner or a seasoned developer new to unit testing, this is the place for you. No prerequisites are needed.
Write it
Learn to write your first unit tests with MSTest. Read what a unit test is, why we need unit tests, and what makes a good unit test. And understand why we shouldn’t try to test private methods.
Identify and fix these four common mistakes when writing your first unit tests. Learn one of these four naming conventions and stick to it. Don’t worry about long test names.
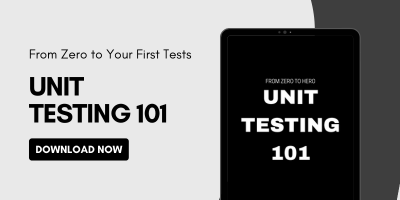
When writing your unit tests, make sure you don’t duplicate logic in Asserts. That’s THE most common mistake in unit testing. Tests should only contain assignments and method calls.
Improve it
Learn how to write good unit tests, avoiding complex setup scenarios and hidden test values.
Make sure to always write a failing test first. And make it fail for the right reasons.
Write tests easy to follow using simple test values.
Use Builders to create test data. And, learn how to write tests that use DateTime.Now.
Strive for a set of always-passing tests, a “Safe Green Zone.” For example, use a culture when parsing numeric strings, instead of relying on a default culture on developers’ machines.
Fake it
Learn what fakes are in unit testing and the difference between stubs and mocks. Follow these tips for better stubs and mocks in C#.
Read how to create fakes with Moq, an easy to use mocking library.
If you find yourself using lots of fakes, take advantage of automocking with TypeBuilder and AutoFixture to write simpler tests.
Master it
Read all the tips from this series on Unit Testing Best Practices.
Deep into assertions, check how to write better assertions and how to write custom assertions.
To see how to put these best practices in place, see how I refactored these real-world tests:
- generating report in a payment system,
- storing and updating OAuth connections,
- removing duplicated email addresses,
- updating email statuses, and,
- speeding up a slow test suite.
If you work with ASP.NET Core, learn how to write tests for HttpClient, Authorization filters, and logging and logging messages.
Happy testing!